For Loop(C++ For Loop Syntax and How For Loop Works? )
Loops are used in programming to repeat a specific block until some end condition is met. There are three types of loops in C++ programming:
- for loop
C++ for Loop Syntax
for(initializationStatement; testExpression; updateStatement) { // codes }
where, only testExpression is mandatory.
How for loop works?
- The initialization statement is executed only once at the beginning.
- Then, the test expression is evaluated.
- If the test expression is false, for loop is terminated. But if the test expression is true, codes inside body of
for
loop is executed and update expression is updated. - Again, the test expression is evaluated and this process repeats until the test expression is false.
Flowchart of for Loop in C++
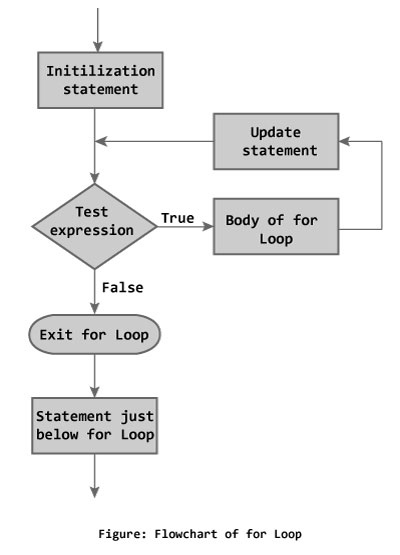
Example 1: C++ for Loop
// C++ Program to find factorial of a number
// Factorial on n = 1*2*3*...*n
#include <iostream>
using namespace std;
int main()
{
int i, n, factorial = 1;
cout << "Enter a positive integer: ";
cin >> n;
for (i = 1; i <= n; ++i) {
factorial *= i; // factorial = factorial * i;
}
cout<< "Factorial of "<<n<<" = "<<factorial;
return 0;
}
OutputEnter a positive integer: 5 Factorial of 5 = 120
Comments
Post a Comment