C++ while Loop(How while loop works?)
In computer programming, loop repeats a certain block of code until some end condition is met.
There are 3 type of loops in C++ Programming:
- while Loop.
C++ while Loop
The syntax of a while loop is:
while (testExpression) { // codes }
where test expression is checked on each entry of the while loop.
How while loop works?
- The while loop evaluates the test expression.
- If the test expression is true, codes inside the body of while loop is evaluated.
- Then, the test expression is evaluated again. This process goes on until the test expression is false.
- When the test expression is false, while loop is terminated.
Flowchart of while Loop
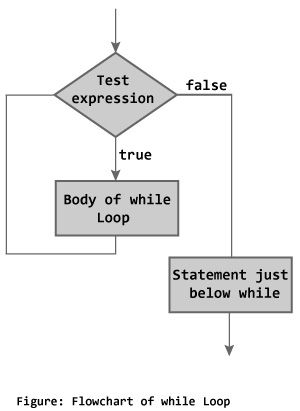
Example 1: C++ while Loop
// C++ Program to compute factorial of a number
// Factorial of n = 1*2*3...*n
#include <iostream>
using namespace std;
int main()
{
int number, i = 1, factorial = 1;
cout << "Enter a positive integer: ";
cin >> number;
while ( i <= number) {
factorial *= i; //factorial = factorial * i;
++i;
}
cout<<"Factorial of "<< number <<" = "<< factorial;
return 0;
}
OutputEnter a positive integer: 4 Factorial of 4 = 24In this program, user is asked to enter a positive integer which is stored in variable number. Let's suppose, user entered 4.Then, the while loop starts executing the code. Here's how while loop works:
- Initially,
i = 1
, test expressioni <= number
is true and factorial becomes 1.- Variable i is updated to 2, test expression is
true
, factorial becomes 2.- Variable i is updated to 3, test expression is
true
, factorial becomes 6.- Variable i is updated to 4, test expression is
true
, factorial becomes 24.- Variable i is updated to 5, test expression is
false
and while loop is terminated.
Comments
Post a Comment