C++ if else(How if else Statement Work?)
C++ if...else
The
if else
executes the codes inside the body of if
statement if the test expression is true and skips the codes inside the body of else
.
If the test expression is false, it executes the codes inside the body of
else
statement and skips the codes inside the body of if
.How the if...else statement works?
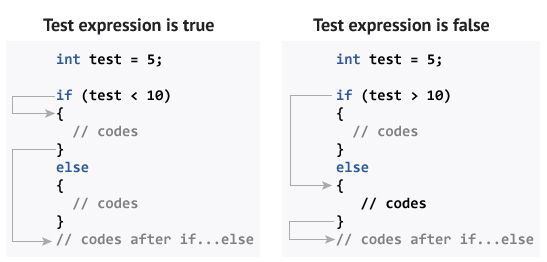
Flowchart of if...else
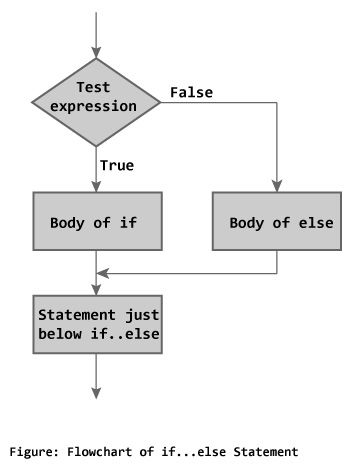
Example 2: C++ if...else Statement
// Program to check whether an integer is positive or negative
// This program considers 0 as positive number
#include <iostream>
using namespace std;
int main()
{
int number;
cout << "Enter an integer: ";
cin >> number;
if ( number >= 0)
{
cout << "You entered a positive integer: " << number << endl;
}
else
{
cout << "You entered a negative integer: " << number << endl;
}
cout << "This line is always printed.";
return 0;
}
Output
Enter an integer: -4 You entered a negative integer: -4. This line is always printed.
Comments
Post a Comment