in C++ How to pass and return an object from a function?
in C++ How to pass and return an object from a function?
How to pass objects to a function?
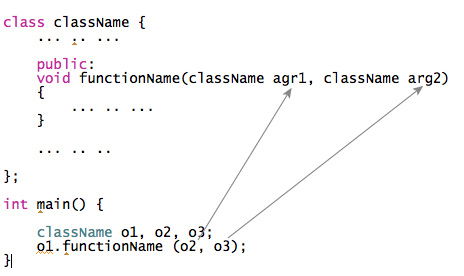
Example 1: Pass Objects to Function
C++ program to add two complex numbers by passing objects to a function.
#include <iostream>
using namespace std;
class Complex
{
private:
int real;
int imag;
public:
Complex(): real(0), imag(0) { }
void readData()
{
cout << "Enter real and imaginary number respectively:"<<endl;
cin >> real >> imag;
}
void addComplexNumbers(Complex comp1, Complex comp2)
{
// real represents the real data of object c3 because this function is called using code c3.add(c1,c2);
real=comp1.real+comp2.real;
// imag represents the imag data of object c3 because this function is called using code c3.add(c1,c2);
imag=comp1.imag+comp2.imag;
}
void displaySum()
{
cout << "Sum = " << real<< "+" << imag << "i";
}
};
int main()
{
Complex c1,c2,c3;
c1.readData();
c2.readData();
c3.addComplexNumbers(c1, c2);
c3.displaySum();
return 0;
}
Output
Enter real and imaginary number respectively: 2 4 Enter real and imaginary number respectively: -3 4 Sum = -1+8i
How to return an object from the function?
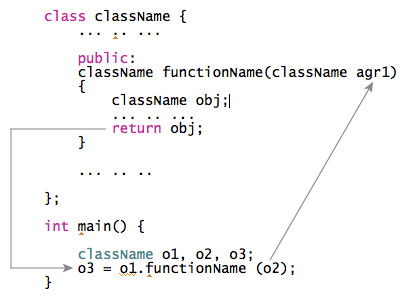
Example 2: Pass and Return Object from the Function
In this program, the sum of complex numbers (object) is returned to the
main()
function and displayed.
#include <iostream>
using namespace std;
class Complex
{
private:
int real;
int imag;
public:
Complex(): real(0), imag(0) { }
void readData()
{
cout << "Enter real and imaginary number respectively:"<<endl;
cin >> real >> imag;
}
Complex addComplexNumbers(Complex comp2)
{
Complex temp;
// real represents the real data of object c3 because this function is called using code c3.add(c1,c2);
temp.real = real+comp2.real;
// imag represents the imag data of object c3 because this function is called using code c3.add(c1,c2);
temp.imag = imag+comp2.imag;
return temp;
}
void displayData()
{
cout << "Sum = " << real << "+" << imag << "i";
}
};
Comments
Post a Comment